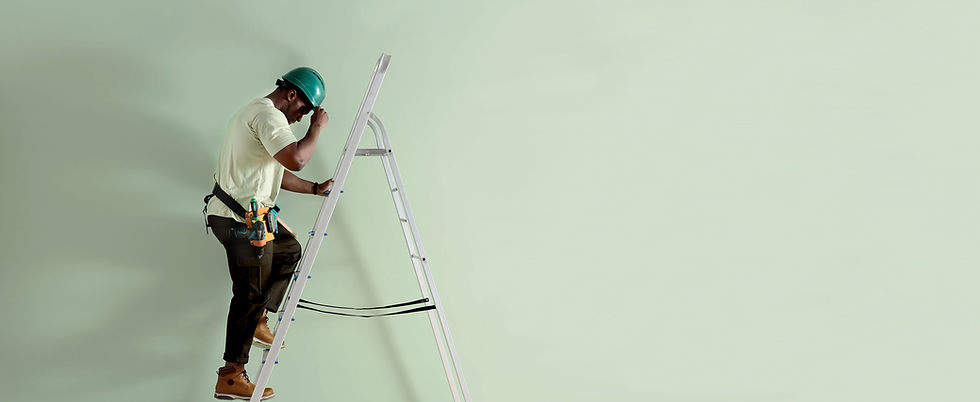
Documentation
As an AI developer, you need to understand and utilize various tools, technologies, and techniques to build effective AI systems. Some of the important resources you should familiarize yourself with include:
-
Programming languages: Python, Java, R, and C++ are some of the most commonly used programming languages for AI development.
-
Deep learning frameworks: TensorFlow, PyTorch, Caffe, and Theano are some popular deep learning frameworks that you can use to build neural networks.
-
Natural language processing (NLP) libraries: spaCy, NLTK, and OpenNLP are some libraries for NLP that you can use to develop AI systems that can understand and generate human language.
-
Computer vision libraries: OpenCV, Pillow, and SimpleCV are some libraries for computer vision that you can use to develop AI systems that can recognize and analyze images and videos.
-
Machine learning libraries: scikit-learn, XGBoost, and LightGBM are some popular machine learning libraries that you can use to build various AI models.
-
Cloud platforms: AWS, Google Cloud, and Microsoft Azure are some cloud platforms that provide AI development tools and services.
-
AI research papers and journals: ArXiv and IEEE Xplore Digital Library are some of the repositories where you can find the latest research papers and journals in AI.
-
Online courses and tutorials: Coursera, Udemy, and edX are some of the platforms where you can find online courses and tutorials to learn AI development.
By familiarizing yourself with these resources, you can build a strong foundation for AI development and keep up with the latest advancements in the field. and work with Keshcutai.
it's important to have clear and comprehensive documentation for your projects. Good documentation helps others understand your code, use it correctly, and contribute to its development. Here are some guidelines for writing effective AI development documentation:
-
Introduction: Start with an overview of the project, including its purpose, target audience, and key features.
-
Installation instructions: Explain how to set up the environment and install the necessary libraries and tools.
-
Usage instructions: Provide clear and concise instructions on how to use the code, including any command-line arguments or configuration files.
-
Data format: Describe the format of the input and output data, including any required data preprocessing steps.
-
Algorithm description: Explain the underlying algorithm, including its assumptions, strengths, and limitations.
-
Technical details: Provide details on the implementation, including any important design decisions and trade-offs.
-
Examples: Include examples of how the code can be used, along with expected results.
-
Troubleshooting: List common problems and their solutions, along with a description of any known limitations.
-
Future work: Outline any planned improvements or features for future releases.
-
Contributions: Explain how others can contribute to the project, including guidelines for submitting bug reports and pull requests.
Ex-
Here is an example of code for an AI developer, in Python. This code implements a simple neural network for image classification using the popular deep learning library, TensorFlow:
-
import tensorflow as tf
from tensorflow import keras -
# Load the MNIST dataset
(x_train, y_train), (x_test, y_test) = keras.datasets.mnist.load_data() -
# Preprocess the data
x_train = x_train.reshape(-1, 28 * 28) / 255.0
x_test = x_test.reshape(-1, 28 * 28) / 255.0 -
# Define the model
model = keras.models.Sequential([
keras.layers.Dense(128, activation='relu', input_shape=(28 * 28,)),
keras.layers.Dense(10, activation='softmax'),
]) -
# Compile the model
model.compile(loss='sparse_categorical_crossentropy',
optimizer='adam',
metrics=['accuracy']) -
# Train the model
model.fit(x_train, y_train, epochs=5) -
# Evaluate the model
test_loss, test_acc = model.evaluate(x_test, y_test)
print('Test accuracy:', test_acc)
This code uses the tensorflow library to load the MNIST dataset, which consists of handwritten digits, and trains a simple neural network to classify them. The model consists of two dense layers with 128 and 10 neurons respectively, with ReLU activation for the first layer and softmax activation for the second. The model is then compiled using the Adam optimizer and sparse categorical crossentropy loss, and trained for 5 epochs on the training data. Finally, the model is evaluated on the test data and its accuracy is printed.